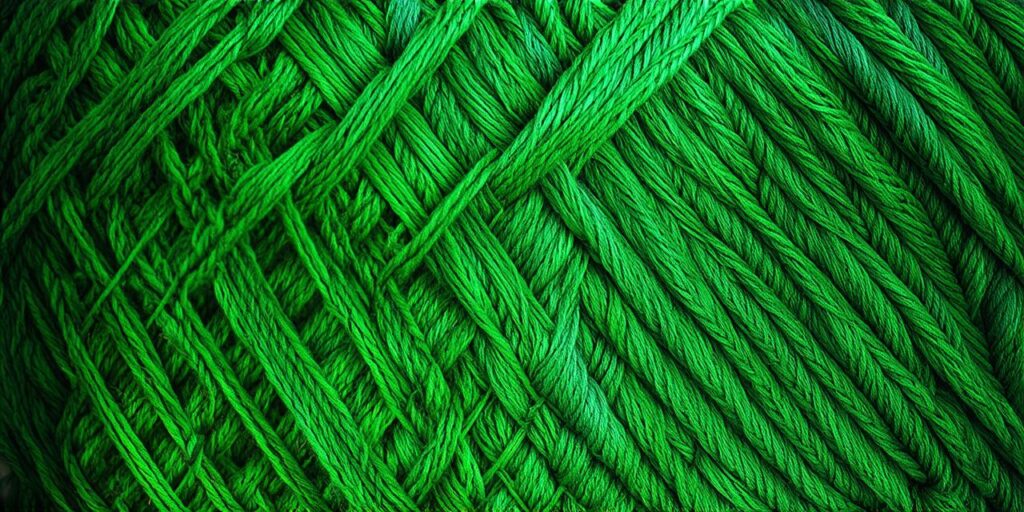
Modifying textures at runtime can be a useful feature for games or other applications that need to change the appearance of objects on-the-fly. However, modifying textures in real time can also be resource-intensive and may cause performance issues if not done carefully.
In this article, we will explore how to modify textures at runtime using threads and best practices to ensure smooth and efficient execution.
Threads are a powerful tool for performing tasks asynchronously in parallel. By leveraging threads, we can modify textures without blocking the main thread of our application and keep it responsive to user input.
Here are some steps to modify textures at runtime using threads:
- Create a texture object and load it into memory. This can be done using the appropriate APIs for your programming language and platform. For example, in C++ and OpenGL, we can use glGenTextures() and glBindTexture() to create and bind textures to a specific slot.
- Create a thread that will modify the texture object. This thread should be responsible for performing any necessary calculations or updates to the texture data.
- Update the thread with new data as needed. This can be done using synchronization mechanisms such as mutexes, semaphores, or atomic variables to ensure that changes are made atomically and safely.
- Once the thread has completed modifying the texture object, it should signal the main thread to rebind the updated texture. This can be done using a signaling mechanism such as a condition variable.
- Finally, rebind the modified texture to the appropriate slot in the render pipeline of your application. This will ensure that any objects that use this texture are updated with the new data.
One important consideration when modifying textures at runtime is the potential for memory fragmentation. As you modify textures, you may need to resize or allocate new chunks of memory to accommodate changes in size or format. To avoid this issue, it’s recommended to use a texture atlas or other techniques that minimize the number of individual textures being used.
Another consideration is the potential for synchronization issues if multiple threads are modifying the same texture object simultaneously. To avoid conflicts and ensure atomicity, it’s important to carefully design your synchronization mechanisms and test your code thoroughly before deploying it to a production environment.
In conclusion, modifying textures at runtime using threads can be a powerful tool for improving the appearance of objects in games or other applications. By following best practices and careful design, we can ensure smooth and efficient execution while avoiding potential issues such as memory fragmentation and synchronization conflicts.