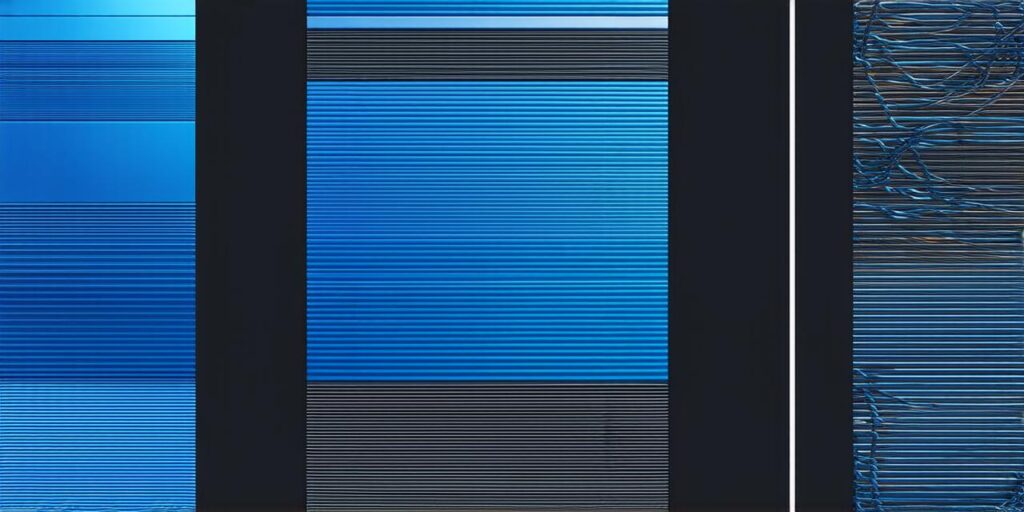
Adding an Editor to Your Unity Project
If you’re looking to add an editor to your Unity project, there are a few options available to you. In this article, we’ll take a look at how you can include an editor in Unity using different methods.
Method 1: Adding an Editor Script
One of the most common ways to add an editor to your Unity project is by creating a custom script and attaching it to the GameObject that you want to edit. This script will allow you to create custom inspectors and editors for that GameObject, which can be accessed in the Unity Editor.
Step 1:
Create a new script in your project by right-clicking on the “Assets” folder in the Project window and selecting “Create” > “C Script”.
Step 2:
Open the script and add the following code to create a new editor for the GameObject:
Method 1: Adding an Editor Script
One of the most common ways to add an editor to your Unity project is by creating a custom script and attaching it to the GameObject that you want to edit. This script will allow you to create custom inspectors and editors for that GameObject, which can be accessed in the Unity Editor.
Step 1:
Create a new script in your project by right-clicking on the “Assets” folder in the Project window and selecting “Create” > “C Script”.
Step 2:
Open the script and add the following code to create a new editor for the GameObject:
csharp
using UnityEngine;
public class MyEditor : EditorWindow
{
private MyGameObject gameObject;
[MenuItem(“My Menu/Show My Editor”)]
static void ShowEditor()
{
MyEditor window = (MyEditor)EditorWindow.GetWindow(typeof(MyEditor), “My Editor”, false, 600, 400);
window.gameObject = Selection.activeGameObject;
}
private void OnGUI()
{
// Add your custom editor here
}
}
[SelectionBase]
public class MyGameObject : GameObject
{
// Your game object properties and methods go here
}
Method 2: Using an Existing Editor Script
Another way to include an editor in Unity is by using an existing script, such as the built-in “ScriptableObject” or “Component”. These scripts allow you to add properties and methods that can be accessed and modified by the Unity Editor.
Method 3: Using an External Editor Plugin
If you want to use an external editor plugin to include an editor in your Unity project, there are a few options available to you. These plugins allow you to create custom inspectors and editors that can be accessed from within the Unity Editor.