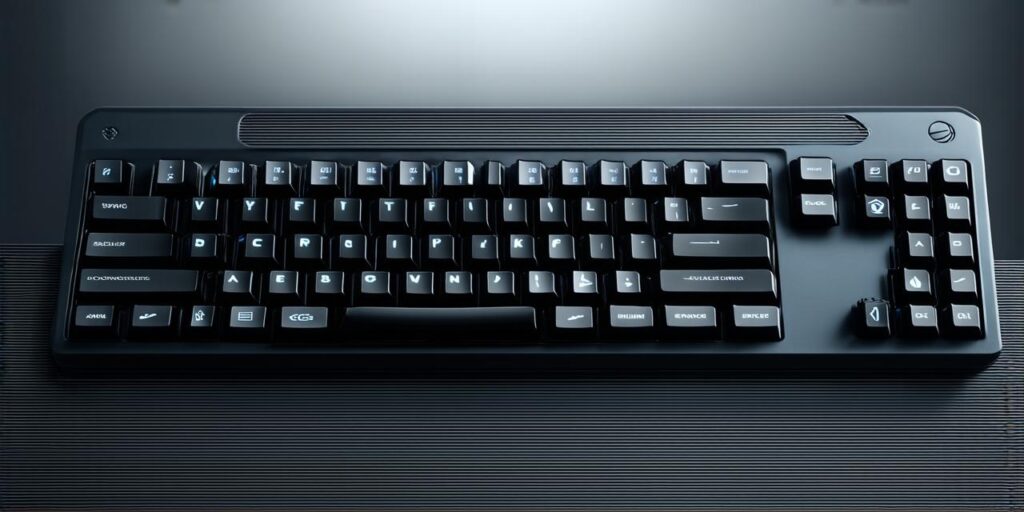
When creating a game or interactive experience in Unity, it is important to be able to capture user input in order to control the behavior of your application.
One way to capture input is by using keys on the keyboard. In this article, we will discuss how to capture input from a key in Unity.
The first step is to determine which key you want to capture input for. For example, if you want to allow the user to move their character forward, you would use the WASD keys (W for up, A for left, S for down, D for right). To capture input from a specific key, you will need to use a script that listens for key presses and responds accordingly.
To create a script that captures input from a key in Unity, you can use the following code as a starting point:
csharp
public class KeyInput : MonoBehaviour
{
public float speed = 10f; // The speed at which the character moves
private Vector3 movement; // A vector to store the movement input
void Update()
{
// Check for key presses
if (Input.GetKeyDown(KeyCode.W) && transform.position.y > 0)
movement = Vector3.up speed Time.deltaTime;
else if (Input.GetKeyDown(KeyCode.S) && transform.position.y < -10)
movement = Vector3.down speed Time.deltaTime;
else if (Input.GetKeyDown(KeyCode.A) && transform.position.x > 10)
movement = Vector3.left speed Time.deltaTime;
else if (Input.GetKeyDown(KeyCode.D) && transform.position.x < -10)
movement = Vector3.right speed Time.deltaTime;
// Apply the movement input to the character
transform.position += movement;
}
}
In this example, we have created a script called “KeyInput” that listens for key presses on the WASD keys and applies movement to the character based on those presses. The speed of the character is controlled by the “speed” variable, which can be adjusted as needed.
To use this script in your game, you will need to attach it to a GameObject (such as a character) and then assign that GameObject to a new script component in the Inspector window. Once you have attached the script, you can adjust the key bindings by changing the values of the KeyCode constants.
In addition to using keys to control movement, there are many other ways to capture input in Unity. You can use a variety of controllers and peripherals, such as joysticks, gamepads, and touchscreens, to control your application. By understanding how to capture input from different devices, you can create more interactive and engaging experiences for your users.