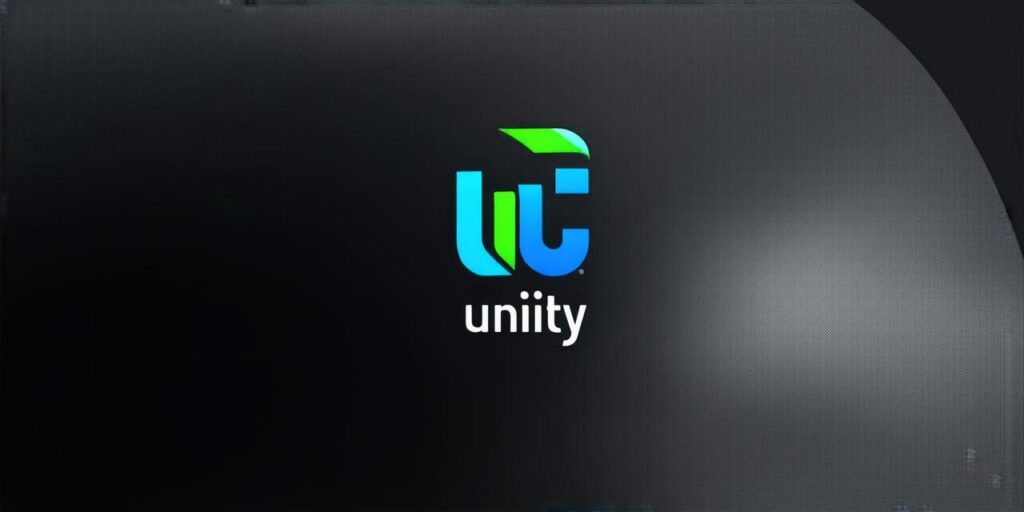
Corrected HTML code:
In this article, we will explore how to develop a script for an editor in Unity.
As an editor script, it allows you to create customized editors that can be used by other developers on your team.
Why Create an Editor Script?
There are several reasons why you may want to create an editor script in Unity:
- Customization: An editor script allows you to create customized editors that meet the specific needs of your team or project. This can help streamline development and improve efficiency.
- Reusability: Once you have created an editor script, it can be reused across multiple projects, saving you time and effort in the long run.
- Integration: An editor script can be integrated with other tools and workflows, making it easier to work with your team and manage your project.
The Basics of an Editor Script
An editor script is a C script that extends the Unity Editor class. This class provides access to the Unity editor’s features and functionality, such as inspectors, selection, and layout.
- Create a new C script in your project.
- Open the script and add the following using statement at the top of the file:
csharp
using UnityEngine;
csharp
public class MyEditor : EditorWindow
{
// Your custom editor logic will go here
}
- Implement your custom editor logic in the MyEditor class. This will include creating a window, setting up an inspector, and adding any other necessary features to your editor.
Customizing Your Editor
Once you have created your editor script, you can customize it to meet your specific needs. Here are some ways to do this:
- Create a new window: You can create a new window for your editor by creating an instance of the EditorWindow class and setting its properties. For example:
csharp
var myWindow = (EditorWindow)EditorWindow.GetWindow(typeof(MyEditor), "My Editor");
- Set up an inspector: You can set up an inspector for your editor by creating a new field in your MyEditor class and using the @Editor attribute to specify its type and layout. For example:
csharp
[Range(0, 1)]
public float myFloat;
[Slider(range new SliderRange(0, 1))]
public void OnGUI()
{
EditorGUILayout.BeginHorizontal();
myFloat = EditorGUILayout.Slider("My Float", myFloat);
EditorGUILayout.EndHorizontal();
}
- Add custom buttons: You can add custom buttons to your editor by creating a new button in your OnGUI function and specifying its properties. For example:
csharp
[Button("My Button")]
public void MyFunction()
{
// Your custom logic goes here
}
Conclusion
Developing an editor script for Unity can be a powerful tool for improving development and efficiency in your team. By following the basics outlined above and customizing your editor to meet your specific needs, you can create a streamlined and effective development workflow.